Python深入教学
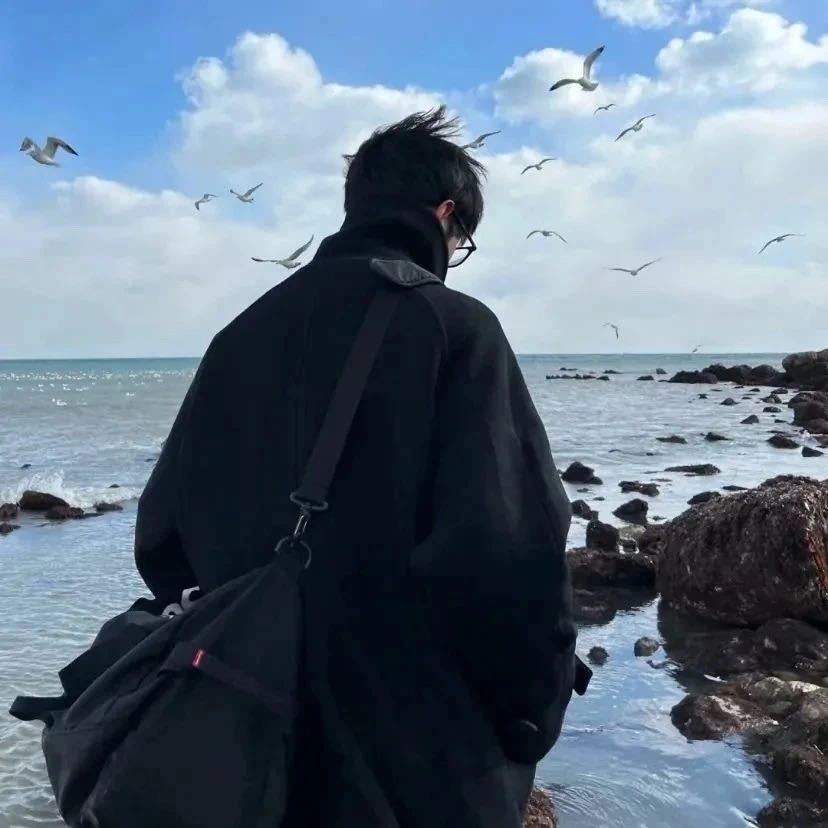
Python深入教学
点我刷新深入学习 Python:从基础到高级的全方位指南
序言
Python 已经成为当今最流行的编程语言之一,因其简洁的语法和强大的功能,被广泛应用于数据科学、人工智能、Web 开发、自动化等多个领域。不论你是编程新手还是有经验的开发者,这篇博文将帮助你更深入地理解 Python,从基础概念到高级应用,全面覆盖。
一、Python 基础知识
1.1 Python 简介
Python 是由 Guido van Rossum 于 1991 年发布的高级编程语言。它以清晰、易读的语法著称,允许开发者用更少的代码实现复杂的功能。
1.2 Python 版本
- Python 2 vs Python 3:虽然 Python 2 仍然存在,但 Python 3 是当前的主流版本,推荐新项目使用 Python 3。Python 3 引入了许多新特性和改进,不向后兼容 Python 2。
1.3 Python 基本语法
变量和数据类型:Python 支持多种数据类型,如整数、浮点数、字符串、列表、元组、字典等。
1
2
3x = 10 # 整数
y = 3.14 # 浮点数
name = "Alice" # 字符串条件语句:
1
2
3
4
5
6if x > 5:
print("x is greater than 5")
elif x == 5:
print("x is 5")
else:
print("x is less than 5")循环:
1
2
3
4
5
6for i in range(5):
print(i)
while x > 0:
print(x)
x -= 1函数:
1
2
3
4def greet(name):
return f"Hello, {name}!"
print(greet("Alice"))
二、Python 进阶概念
2.1 面向对象编程(OOP)
Python 是一门面向对象的语言,这意味着你可以创建和使用类和对象来组织代码。
类和对象:
1
2
3
4
5
6
7
8
9
10class Dog:
def __init__(self, name, age):
self.name = name
self.age = age
def bark(self):
return f"{self.name} says woof!"
my_dog = Dog("Buddy", 3)
print(my_dog.bark())继承与多态:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
return "Woof!"
class Cat(Animal):
def speak(self):
return "Meow!"
animals = [Dog(), Cat()]
for animal in animals:
print(animal.speak())
2.2 Python 内置函数与标准库
Python 内置了许多有用的函数和模块,极大地提高了开发效率。
内置函数:如
len()
,type()
,range()
,print()
等。1
2
3my_list = [1, 2, 3]
print(len(my_list)) # 输出 3
print(type(my_list)) # 输出 <class 'list'>标准库:Python 标准库涵盖了许多常用的模块,如
os
,sys
,math
,datetime
,json
等。1
2
3
4
5import os
print(os.getcwd()) # 获取当前工作目录
import math
print(math.sqrt(16)) # 计算平方根
三、Python 高级应用
3.1 异常处理
在编写代码时,处理可能出现的错误是非常重要的,Python 提供了异常处理机制来处理这些情况。
- try-except 语句:
1
2
3
4
5
6try:
result = 10 / 0
except ZeroDivisionError:
print("You can't divide by zero!")
finally:
print("This will always execute.")
3.2 文件操作
Python 提供了简单的接口来读写文件。
读取文件:
1
2
3with open('example.txt', 'r') as file:
content = file.read()
print(content)写入文件:
1
2with open('example.txt', 'w') as file:
file.write("Hello, World!")
3.3 生成器与迭代器
生成器和迭代器是 Python 中非常强大的工具,尤其适用于处理大量数据时。
生成器:
1
2
3
4
5
6
7def my_generator():
for i in range(5):
yield i
gen = my_generator()
for value in gen:
print(value)迭代器:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17class MyIterator:
def __init__(self, max_value):
self.current = 0
self.max_value = max_value
def __iter__(self):
return self
def __next__(self):
if self.current < self.max_value:
self.current += 1
return self.current
else:
raise StopIteration
for num in MyIterator(5):
print(num)
四、Python 应用场景
4.1 Web 开发
Python 有多种流行的 Web 框架,如 Django、Flask、FastAPI 等,可以快速构建和部署 Web 应用。
- Flask 示例:
1
2
3
4
5
6
7
8
9
10from flask import Flask
app = Flask(__name__)
def hello_world():
return 'Hello, World!'
if __name__ == '__main__':
app.run(debug=True)
4.2 数据科学与机器学习
Python 是数据科学和机器学习领域的首选语言,有丰富的库支持,如 NumPy、Pandas、Matplotlib、Scikit-learn、TensorFlow 和 PyTorch。
数据分析示例:
1
2
3
4
5import pandas as pd
data = {'Name': ['Alice', 'Bob', 'Charlie'], 'Age': [24, 30, 22]}
df = pd.DataFrame(data)
print(df.describe())机器学习示例:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15from sklearn.datasets import load_iris
from sklearn.model_selection import train_test_split
from sklearn.ensemble import RandomForestClassifier
# 加载数据
iris = load_iris()
X_train, X_test, y_train, y_test = train_test_split(iris.data, iris.target, test_size=0.3)
# 创建模型并训练
clf = RandomForestClassifier()
clf.fit(X_train, y_train)
# 预测
predictions = clf.predict(X_test)
print(predictions)
4.3 自动化脚本
Python 非常适合编写自动化脚本,例如自动化任务、文件管理、网络爬虫等。
- 网络爬虫示例:
1
2
3
4
5
6
7
8import requests
from bs4 import BeautifulSoup
response = requests.get('https://www.example.com')
soup = BeautifulSoup(response.content, 'html.parser')
for link in soup.find_all('a'):
print(link.get('href'))
五、Python 的优化与最佳实践
5.1 代码优化
优化代码不仅能提高程序性能,还能提升可读性和可维护性。
使用列表推导:
1
squares = [x**2 for x in range(10)]
使用生成器表达式:
1
gen = (x**2 for x in range(10))
使用内置函数:如
map()
,filter()
,reduce()
等。
5.2 编写高效的代码
- 避免不必要的计算:缓存结果,避免重复计算。
- 使用多线程或多进程:处理 I/O 密集型任务时,使用
threading
或multiprocessing
模块来提高性能。 - 合理使用内存:避免在内存中保留大量数据,使用生成器等惰性求值技术。
六、Python 社区与资源
Python 社区活跃,有大量的在线资源、教程、论坛和开源项目可供学习和借鉴。
- 官方网站:Python 官方网站(python.org)提供了丰富的文档和教程。
- PyPI:Python Package Index 是一个巨大的第三方库资源库,可以帮助你快速找到所需的库。
- 在线学习平台:如 Coursera、Udemy、edX、LeetCode 提供了大量的 Python 课程。
总结
Python 作为一门功能强大的编程语言,应用范围广泛,无论是初学者还是资深
开发者,都能在其中找到适合自己的发展方向。通过这篇博客,你应该对 Python 的基础知识、进阶概念、高级应用以及优化技巧有了全面的了解。希望你能在 Python 的世界中不断探索,提升自己的编程能力。
如果你有任何疑问或需要更多的示例和解释,欢迎随时联系我!
你对这个主题有什么特别的兴趣或问题吗?